Beginners guide to learn shell scripting basics of If statement and for. while loops. The article includes small scripts of if, for and while loop.
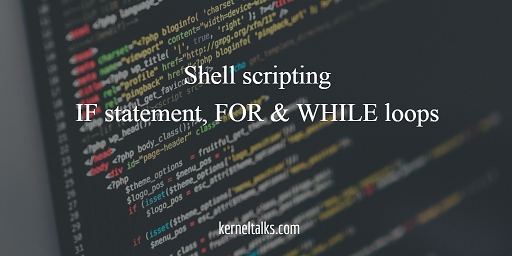
This article can be referred to as a beginner’s guide to the introduction of shell scripting. We will be discussing various loops that are used in shell or bash scripting. In this chapter, we will discuss on if, for and while loop of scripting: if statement, for loop and while loop.
IF statement
If the loop is a conditional loop in scripting. It is used to check some conditions and perform actions based on the condition being true or false. If the loop structure is :
if [ condition ]
then
<execute this code>
else
<execute this code>
fi
If starts with condition check, if conditions match then it will execute the following code (after then). If the condition is false then it will execute code after else. If a statement can be used without else part too.
Example :
# cat if_statement.sh
#!/bin/bash
if [ $1 -lt 100 ]
then
echo "Your number is smaller than 100"
else
echo "Your number is greater than 100"
fi
# sh if_statement.sh 34
Your number is smaller than 100
If you execute this script, the loop will read the first argument as $1 and compare it with 100. Accordingly it will display output.
Bash FOR loop
Executing the same set of commands for the number of times loops are used in scripting. One of them is for loop. It takes input as a series of values and executes the following code repeatedly for each value. For loop structure is as below :
for i in <values>
do
<execute this code>
done
Here is reads values as a variable i
and then $i
can be used in the following code. Once all the values processed loops stops and script proceed to the next line of code.
Example :
# cat for_loop.sh
#!/bin/bash
for i in 1 2 3 4 5
do
echo First value in the series is $i
done
# sh for_loop.sh
First value in the series is 1
First value in the series is 2
First value in the series is 3
First value in the series is 4
First value in the series is 5
You can see variable $i
is being fed with different values for each run of the loop. Once all values in range processed, the loop exits.
Bash WHILE loop
While is another loop used in programming which runs on condition. It keeps on running until the condition is met. Once the condition is un-matched, it exists. It’s a conditional loop! While loop structure is :
while [ condition ]
do
<execute this code>
done
While loop starts with the condition. If the condition is met it execute the following code and then again goes to verify condition. If the condition is still met then the next iteration of code execution happens. It continues to condition falses.
Example :
# cat while_loop.sh
#!/bin/bash
count=0
while [ $count -lt 3 ]
do
echo Count is $count
count=$(expr $count + 1)
done
# sh while_loop.sh
Count is 0
Count is 1
Count is 2
In the above example we have incremental counter code. We put up a condition for while loop is it can execute till counter less than 3. You can observe output the while loops exist when the counter hits 2.
These are three widely and commonly used for scripting! If you have any suggestions/feedback/corrections for this article please let us know in the comments below.